Intro
Master error handling with 5 essential error trapping functions, including error types, debugging techniques, and exception handling methods to improve code reliability and robustness.
Error trapping is a crucial aspect of programming that involves anticipating, detecting, and handling errors that may occur during the execution of a program. It helps prevent program crashes, ensures data integrity, and provides a better user experience. In this article, we will delve into the world of error trapping functions, exploring their importance, types, and applications.
Error trapping functions are essential in programming because they enable developers to write robust and reliable code. By incorporating error trapping mechanisms, programmers can identify and handle potential errors, reducing the likelihood of program failures and data corruption. Moreover, error trapping functions facilitate debugging, making it easier to identify and fix errors, which in turn, improves the overall quality of the software.
Error trapping functions can be categorized into several types, including input validation, error detection, and exception handling. Input validation involves checking user input to ensure it conforms to expected formats and ranges. Error detection entails identifying errors that occur during program execution, such as division by zero or out-of-range values. Exception handling, on the other hand, involves catching and handling exceptions that are raised during program execution, providing a way to recover from errors and continue executing the program.
Error Trapping Functions Overview
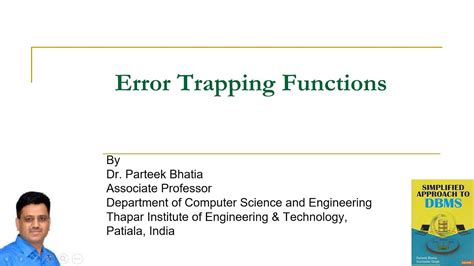
Error trapping functions are used in various programming languages, including Java, Python, C++, and JavaScript. These functions can be used to handle different types of errors, such as syntax errors, runtime errors, and logical errors. By using error trapping functions, developers can write more robust and reliable code, reducing the likelihood of program crashes and data corruption.
Types of Error Trapping Functions

There are several types of error trapping functions, including:
- Try-catch blocks: These blocks are used to catch and handle exceptions that are raised during program execution.
- Error handlers: These are functions that are called when an error occurs, providing a way to recover from the error and continue executing the program.
- Input validation functions: These functions are used to check user input to ensure it conforms to expected formats and ranges.
- Debugging functions: These functions are used to identify and fix errors, providing a way to debug the program.
Error Handling Mechanisms
Error handling mechanisms are used to detect and handle errors that occur during program execution. These mechanisms can be categorized into several types, including: * Exception handling: This involves catching and handling exceptions that are raised during program execution. * Error detection: This involves identifying errors that occur during program execution, such as division by zero or out-of-range values. * Input validation: This involves checking user input to ensure it conforms to expected formats and ranges.Error Trapping Functions in Programming Languages

Error trapping functions are used in various programming languages, including:
- Java: Java provides a built-in exception handling mechanism that allows developers to catch and handle exceptions that are raised during program execution.
- Python: Python provides a try-except block that allows developers to catch and handle exceptions that are raised during program execution.
- C++: C++ provides a try-catch block that allows developers to catch and handle exceptions that are raised during program execution.
- JavaScript: JavaScript provides a try-catch block that allows developers to catch and handle exceptions that are raised during program execution.
Benefits of Error Trapping Functions
Error trapping functions provide several benefits, including: * Improved program reliability: By detecting and handling errors, error trapping functions can improve the reliability of a program. * Reduced program crashes: By catching and handling exceptions, error trapping functions can reduce the likelihood of program crashes. * Better user experience: By providing informative error messages, error trapping functions can improve the user experience. * Easier debugging: By providing detailed error information, error trapping functions can make it easier to debug a program.Best Practices for Using Error Trapping Functions
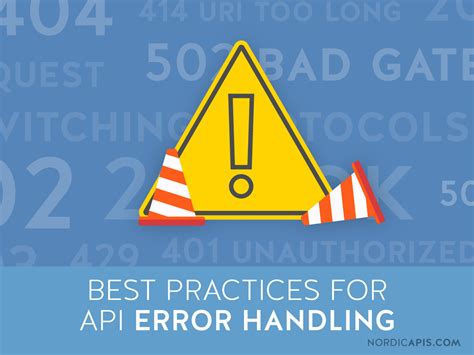
Here are some best practices for using error trapping functions:
- Use try-catch blocks to catch and handle exceptions that are raised during program execution.
- Use error handlers to provide a way to recover from errors and continue executing the program.
- Use input validation functions to check user input to ensure it conforms to expected formats and ranges.
- Use debugging functions to identify and fix errors, providing a way to debug the program.
Common Errors in Error Trapping Functions
Here are some common errors that can occur when using error trapping functions: * Unhandled exceptions: Failing to catch and handle exceptions that are raised during program execution can lead to program crashes. * Incorrect error handling: Handling errors incorrectly can lead to program crashes or data corruption. * Insufficient error information: Failing to provide detailed error information can make it difficult to debug a program.Gallery of Error Trapping Functions
Error Trapping Functions Image Gallery

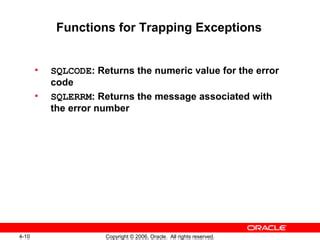








Frequently Asked Questions
What is error trapping?
+Error trapping is a technique used to detect and handle errors that occur during program execution.
Why is error trapping important?
+Error trapping is important because it helps prevent program crashes, ensures data integrity, and provides a better user experience.
How do I use error trapping functions?
+To use error trapping functions, you can use try-catch blocks, error handlers, input validation functions, and debugging functions.
In conclusion, error trapping functions are essential in programming because they enable developers to write robust and reliable code. By incorporating error trapping mechanisms, programmers can identify and handle potential errors, reducing the likelihood of program failures and data corruption. We hope this article has provided you with a comprehensive understanding of error trapping functions and their importance in programming. If you have any questions or comments, please do not hesitate to share them with us. We would be happy to hear from you and provide further guidance on this topic.