Intro
Discover 5 ways variadic templates boost C++ programming with flexible functions, parameter packs, and tuple manipulation, enhancing generic coding and meta-programming techniques.
The concept of variadic templates has revolutionized the way programmers approach generic programming in C++. Introduced in C++11, variadic templates provide a powerful tool for creating functions and classes that can handle a variable number of arguments. This flexibility has opened up new avenues for metaprogramming, type manipulation, and generic algorithm design. In this article, we will delve into the world of variadic templates, exploring their syntax, applications, and the benefits they bring to modern C++ programming.
Variadic templates are a type of template that can accept a variable number of template arguments. This is achieved through the use of a parameter pack, which is a sequence of template parameters that can be expanded at compile-time. The syntax for declaring a variadic template involves the use of an ellipsis (...) in the template parameter list, indicating that the preceding parameter is a pack that can be expanded.
One of the primary advantages of variadic templates is their ability to simplify code and reduce boilerplate. By allowing functions and classes to handle any number of arguments, variadic templates enable programmers to write more generic and reusable code. This not only improves code quality but also enhances maintainability, as changes to the template can automatically propagate to all instantiations.
Introduction to Variadic Templates

To understand variadic templates, it's essential to grasp the basics of template metaprogramming in C++. Templates allow for generic programming by enabling functions and classes to work with different data types without requiring separate implementations for each type. Variadic templates extend this concept by allowing the template parameter list to be variable, enabling more flexible and expressive generic programming.
Parameter Packs and Expansion

Parameter packs are the core mechanism behind variadic templates. A parameter pack is a template parameter that can represent any number of template arguments, including zero. This is denoted by an ellipsis (...) in the template parameter list. When a variadic template is instantiated, the parameter pack is replaced by the actual template arguments provided, allowing the template to adapt to different numbers and types of arguments.
Expanding Parameter Packs
Parameter packs can be expanded using the ...
operator. This operator unpacks the parameter pack, allowing each element to be processed individually. Expansion can occur in various contexts, such as function parameter lists, template argument lists, and even within expressions.
Applications of Variadic Templates
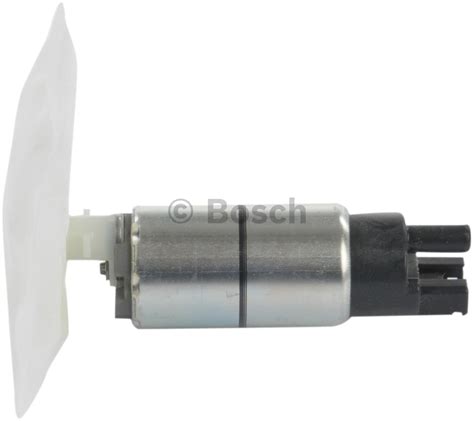
Variadic templates have numerous applications in C++ programming, ranging from simplifying function overloading to implementing complex metaprogramming techniques. Some of the key applications include:
- Generic Functions: Variadic templates enable the creation of generic functions that can handle any number of arguments, making them highly reusable and flexible.
- Type Manipulation: By manipulating parameter packs, developers can perform complex type operations, such as type concatenation, filtering, and transformation.
- Tuple and Variant Implementations: Variadic templates are crucial in the implementation of
std::tuple
andstd::variant
, which are part of the C++ Standard Library.
Implementing a Variadic Template Function
Implementing a variadic template function involves declaring a function template with a parameter pack in its parameter list. The parameter pack can then be expanded within the function body to process each argument individually.
template
void printArguments(Args... args) {
(std::cout << ... << args) << std::endl;
}
This example demonstrates a simple variadic template function printArguments
that takes any number of arguments and prints them to the console. The expression (std::cout << ... << args)
expands the parameter pack args
, inserting std::cout <<
between each argument, effectively printing all arguments.
Best Practices and Considerations
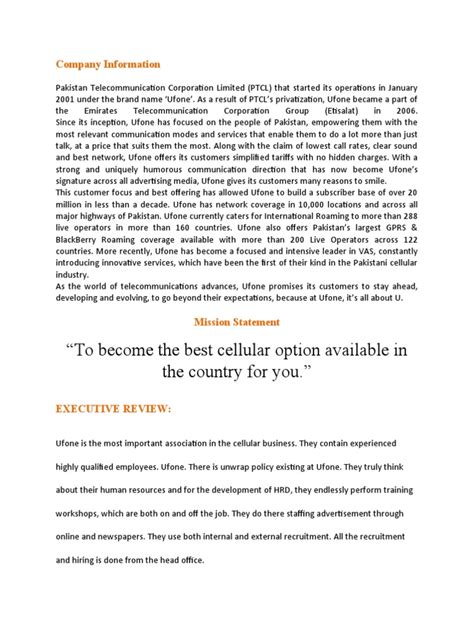
When working with variadic templates, several best practices and considerations can help ensure that the code is efficient, readable, and maintainable:
- SFINAE (Substitution Failure Is Not An Error): Use SFINAE to selectively enable or disable function templates based on the types of arguments provided, enhancing flexibility and preventing compilation errors.
- Parameter Pack Expansion: Master the techniques of expanding parameter packs to manipulate and process arguments effectively.
- Type Traits and Metafunctions: Leverage type traits and metafunctions to perform type manipulation and inspection, which are often necessary when working with variadic templates.
Common Pitfalls and Troubleshooting
Despite their power, variadic templates can lead to complex compilation errors if not used carefully. Common pitfalls include incorrect expansion of parameter packs, failure to handle zero-length parameter packs, and overly complex metaprogramming that can lead to compilation errors or performance issues.
Future Directions and C++ Evolution

The C++ language is continuously evolving, with new features and improvements being added to the standard. Variadic templates, introduced in C++11, have seen enhancements and refinements in subsequent standards, such as C++14 and C++17. Future directions may include further simplifications of template metaprogramming, improved support for concepts (a feature introduced in C++20), and potential extensions to variadic templates to handle even more complex generic programming scenarios.
C++20 and Beyond
C++20 introduces concepts, which are a major extension to the template system, allowing for more expressive and constrained generic programming. While concepts are not a direct extension of variadic templates, they can be used in conjunction with them to create more robust and maintainable generic code.
Variadic Templates Image Gallery
What are variadic templates in C++?
+
Variadic templates are a feature in C++ that allows for functions and classes to handle a variable number of arguments, enhancing generic programming capabilities.
How do parameter packs work in variadic templates?
+
Parameter packs are sequences of template parameters that can be expanded at compile-time, allowing for the manipulation and processing of individual arguments within a variadic template.
What are some common applications of variadic templates?
+
Variadic templates are used in generic functions, type manipulation, and the implementation of tuples and variants, among other applications.
How do I troubleshoot compilation errors related to variadic templates?
+
Troubleshooting involves carefully examining the expansion of parameter packs, ensuring correct handling of zero-length packs, and simplifying complex metaprogramming constructs.
What future developments can we expect for variadic templates in C++?
+
Future developments may include further refinements to template metaprogramming, enhanced support for concepts, and potential extensions to variadic templates for more complex generic programming scenarios.
Variadic Templates Image Gallery


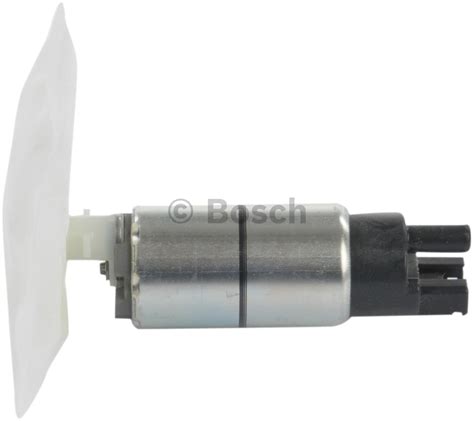
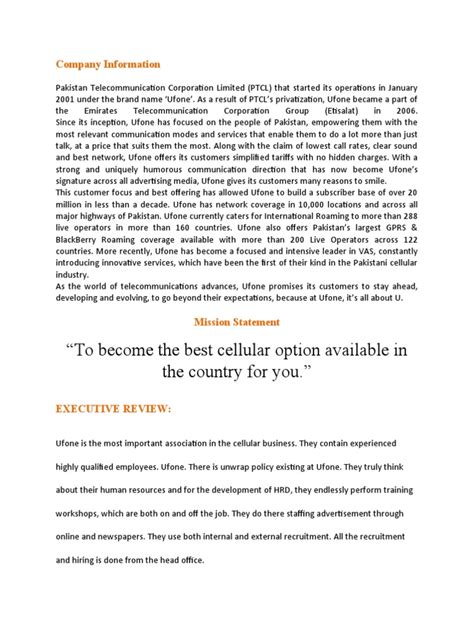





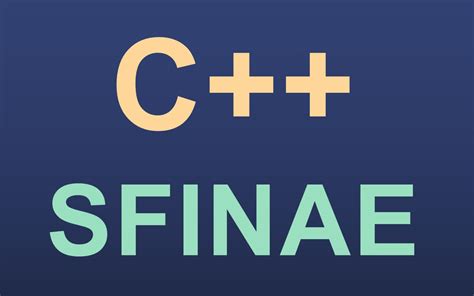
What are variadic templates in C++?
+Variadic templates are a feature in C++ that allows for functions and classes to handle a variable number of arguments, enhancing generic programming capabilities.
How do parameter packs work in variadic templates?
+Parameter packs are sequences of template parameters that can be expanded at compile-time, allowing for the manipulation and processing of individual arguments within a variadic template.
What are some common applications of variadic templates?
+Variadic templates are used in generic functions, type manipulation, and the implementation of tuples and variants, among other applications.
How do I troubleshoot compilation errors related to variadic templates?
+Troubleshooting involves carefully examining the expansion of parameter packs, ensuring correct handling of zero-length packs, and simplifying complex metaprogramming constructs.
What future developments can we expect for variadic templates in C++?
+Future developments may include further refinements to template metaprogramming, enhanced support for concepts, and potential extensions to variadic templates for more complex generic programming scenarios.
In conclusion, variadic templates are a powerful tool in modern C++ programming, offering unparalleled flexibility and expressiveness in generic programming. By mastering variadic templates, developers can create more robust, reusable, and maintainable code, leveraging the full potential of C++'s template metaprogramming capabilities. Whether you're a seasoned C++ developer or just starting your journey, understanding and applying variadic templates can significantly enhance your programming skills and the quality of your code. We invite you to share your experiences, ask questions, and explore the vast possibilities that variadic templates have to offer in the world of C++ programming.