Intro
Discover 5 ways Docker sends emails, leveraging containerization, email services, and automation tools for efficient communication, using SMTP, APIs, and more.
Docker has revolutionized the way we develop, deploy, and manage applications. One of the essential features of many applications is the ability to send emails. Whether it's for user notifications, password reset instructions, or automated reports, email sending is a critical functionality. In this article, we will explore five ways Docker can be used to send emails.
Sending emails from a Docker container can be achieved through various methods, including using a mail server, a third-party email service, or a custom email sending script. Each method has its advantages and disadvantages, and the choice of which one to use depends on the specific requirements of the application. In the following sections, we will delve into the details of each method and provide examples of how to implement them.
Introduction to Docker and Email Sending
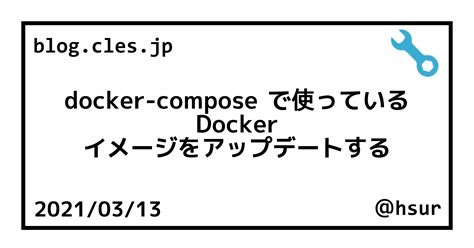
Before we dive into the methods of sending emails from Docker, it's essential to understand the basics of Docker and how it can be used to send emails. Docker provides a lightweight and portable way to deploy applications, and it can be used to send emails by installing a mail server or using a third-party email service. In this section, we will provide an overview of Docker and its features, as well as the basics of email sending.
Method 1: Using a Mail Server
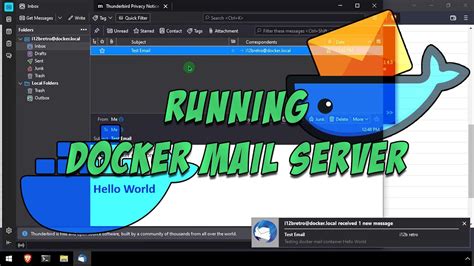
One of the most common methods of sending emails from a Docker container is by using a mail server. A mail server is a software application that handles email sending and receiving. There are several mail servers available, including Postfix, Sendmail, and Exim. In this section, we will provide an example of how to use Postfix to send emails from a Docker container.
To use Postfix, you need to install it in your Docker container and configure it to send emails. You can do this by creating a Dockerfile that installs Postfix and configures it to send emails. Here is an example of a Dockerfile that installs Postfix:
FROM ubuntu:latest
RUN apt-get update && apt-get install -y postfix
Once you have installed Postfix, you need to configure it to send emails. You can do this by creating a configuration file that specifies the mail server settings. Here is an example of a configuration file that specifies the mail server settings:
sudo postconf -e "mydestination = example.com"
sudo postconf -e "mynetworks = 127.0.0.0/8 [::ffff:127.0.0.0]/104 [::1]/128"
sudo postconf -e "inet_interfaces = all"
sudo postconf -e "inet_protocols = all"
sudo postconf -e "relayhost = "
Once you have configured Postfix, you can use it to send emails from your Docker container. You can do this by using the mail
command to send an email. Here is an example of how to use the mail
command to send an email:
echo "Hello World" | mail -s "Test Email" example@example.com
Method 2: Using a Third-Party Email Service
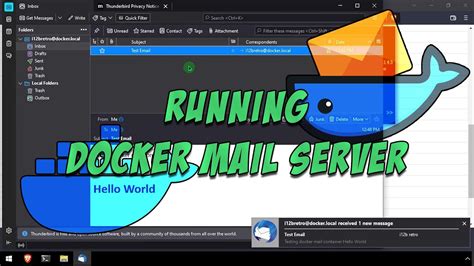
Another method of sending emails from a Docker container is by using a third-party email service. There are several email services available, including SendGrid, Mailgun, and Amazon SES. In this section, we will provide an example of how to use SendGrid to send emails from a Docker container.
To use SendGrid, you need to create an account and obtain an API key. You can then use the API key to send emails from your Docker container. Here is an example of how to use the SendGrid API to send an email:
import os
from sendgrid import SendGridAPIClient
from sendgrid.helpers.mail import Mail
message = Mail(
from_email='example@example.com',
to_emails='example@example.com',
subject='Test Email',
plain_text_content='Hello World'
)
try:
sg = SendGridAPIClient(os.environ.get('SENDGRID_API_KEY'))
response = sg.send(message)
print(response.status_code)
print(response.body)
print(response.headers)
except Exception as e:
print(e)
Method 3: Using a Custom Email Sending Script
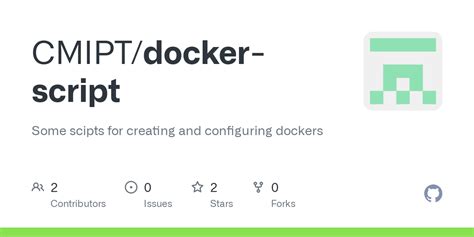
Another method of sending emails from a Docker container is by using a custom email sending script. You can write a script in a programming language such as Python or Node.js that sends an email using a mail server or a third-party email service. In this section, we will provide an example of how to use a Python script to send an email using a mail server.
To use a custom email sending script, you need to write a script that sends an email using a mail server or a third-party email service. Here is an example of a Python script that sends an email using a mail server:
import smtplib
from email.mime.text import MIMEText
msg = MIMEText('Hello World')
msg['Subject'] = 'Test Email'
msg['From'] = 'example@example.com'
msg['To'] = 'example@example.com'
server = smtplib.SMTP('smtp.example.com', 587)
server.starttls()
server.login('example@example.com', 'password')
server.sendmail('example@example.com', 'example@example.com', msg.as_string())
server.quit()
Method 4: Using a Docker Container with a Mail Server

Another method of sending emails from a Docker container is by using a Docker container with a mail server. You can create a Docker container that runs a mail server, such as Postfix, and use it to send emails. In this section, we will provide an example of how to create a Docker container with a mail server.
To create a Docker container with a mail server, you need to create a Dockerfile that installs the mail server and configures it to send emails. Here is an example of a Dockerfile that installs Postfix:
FROM ubuntu:latest
RUN apt-get update && apt-get install -y postfix
Once you have created the Dockerfile, you can build the Docker image and run the Docker container. Here is an example of how to build the Docker image and run the Docker container:
docker build -t my-mail-server .
docker run -d -p 25:25 my-mail-server
Once you have run the Docker container, you can use it to send emails. You can do this by using the mail
command to send an email. Here is an example of how to use the mail
command to send an email:
echo "Hello World" | mail -s "Test Email" example@example.com
Method 5: Using a Docker Compose File
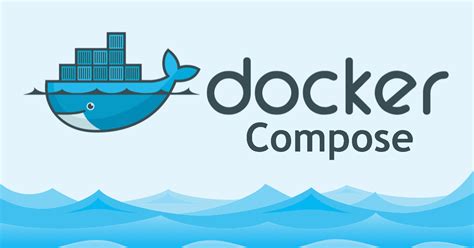
Another method of sending emails from a Docker container is by using a Docker Compose file. You can create a Docker Compose file that defines a service that sends emails using a mail server or a third-party email service. In this section, we will provide an example of how to create a Docker Compose file that defines a service that sends emails using a mail server.
To create a Docker Compose file, you need to define a service that sends emails using a mail server or a third-party email service. Here is an example of a Docker Compose file that defines a service that sends emails using a mail server:
version: '3'
services:
mail-server:
image: postfix
ports:
- "25:25"
environment:
- MYDESTINATION=example.com
- MYNETWORKS=127.0.0.0/8 [::ffff:127.0.0.0]/104 [::1]/128
- INET_INTERFACES=all
- INET_PROTOCOLS=all
- RELAYHOST=
Once you have created the Docker Compose file, you can use it to start the service and send emails. Here is an example of how to start the service and send an email:
docker-compose up -d
echo "Hello World" | mail -s "Test Email" example@example.com
Email Sending Gallery
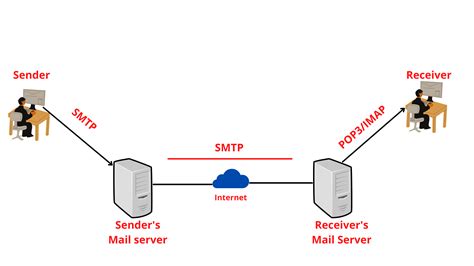
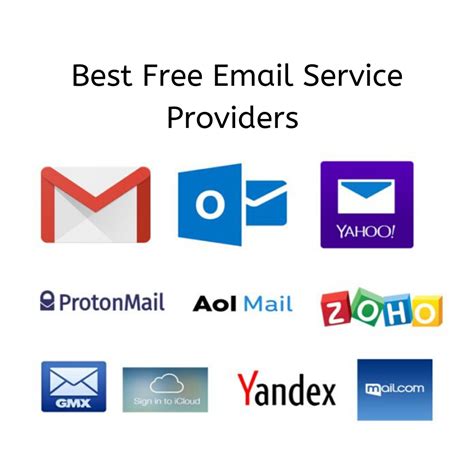
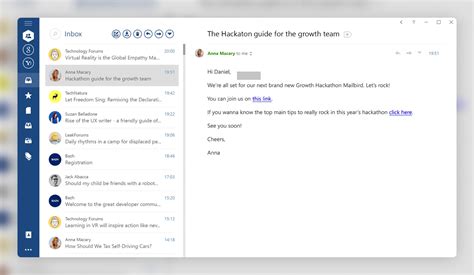
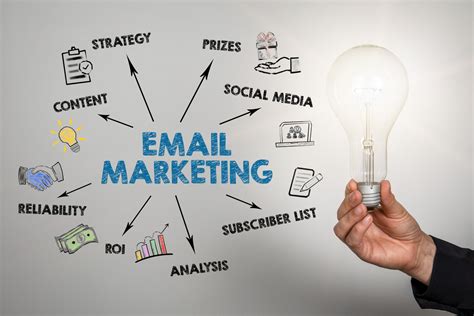
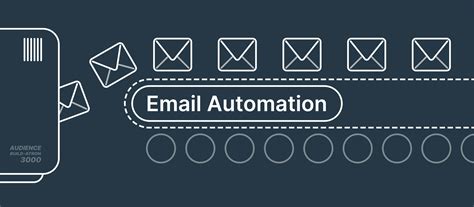
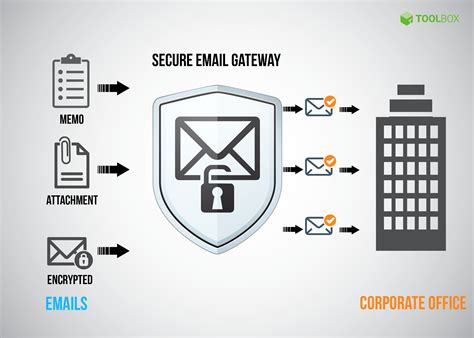
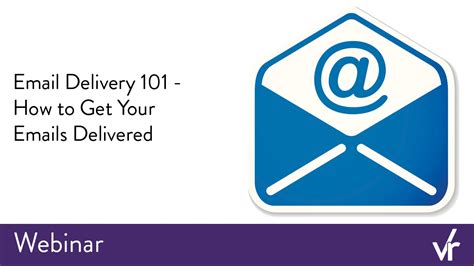
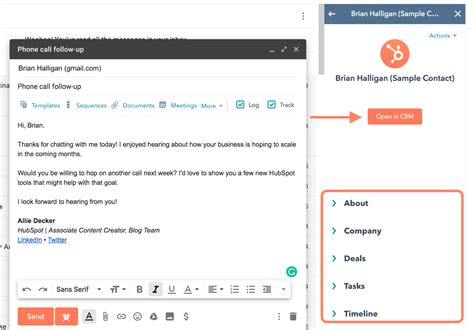
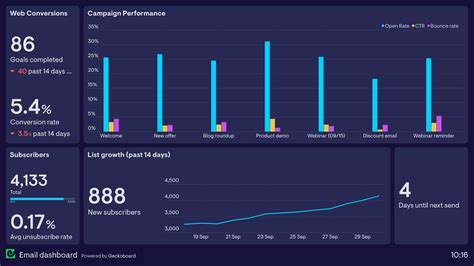
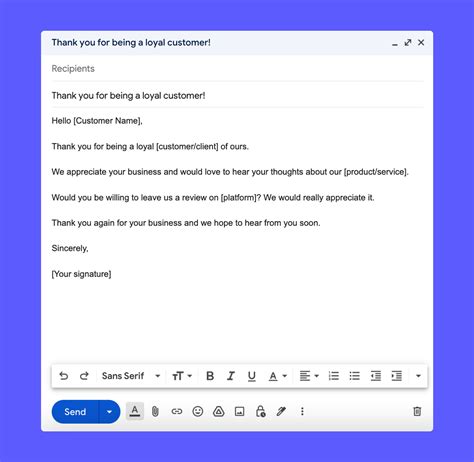
What is the best way to send emails from a Docker container?
+The best way to send emails from a Docker container depends on the specific requirements of the application. You can use a mail server, a third-party email service, or a custom email sending script.
How do I configure Postfix to send emails from a Docker container?
+To configure Postfix to send emails from a Docker container, you need to create a configuration file that specifies the mail server settings. You can then use the `postconf` command to configure Postfix.
What is the difference between a mail server and a third-party email service?
+A mail server is a software application that handles email sending and receiving, while a third-party email service is a cloud-based service that provides email sending and receiving capabilities. Mail servers are typically more customizable, but require more maintenance, while third-party email services are easier to use, but may have limitations on customization.
In