Intro
Master Djangos template if statements with this comprehensive guide, covering conditional tags, template logic, and best practices for dynamic web development with Django templates.
Django template if statements are a crucial part of building dynamic web applications. They allow you to control the flow of your template based on conditions, making your web pages more interactive and user-friendly. In this article, we will delve into the world of Django template if statements, exploring their syntax, usage, and best practices.
The importance of if statements in Django templates cannot be overstated. They enable you to make decisions based on the data passed to the template from the view, allowing you to display or hide content, perform actions, and even loop through data. With if statements, you can create complex and dynamic web pages that adapt to different scenarios, making your application more engaging and efficient.
In Django, the template engine is designed to be simple and easy to use, yet powerful enough to handle complex logic. The if statement is a fundamental part of this engine, and it's used extensively in Django development. Whether you're building a simple blog or a complex e-commerce site, understanding how to use if statements in Django templates is essential for creating a dynamic and interactive user experience.
Basic Syntax of If Statements in Django Templates
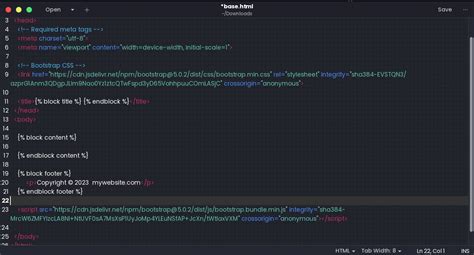
The basic syntax of an if statement in a Django template is straightforward. You start with the {% if %}
tag, followed by the condition you want to evaluate, and then the {% endif %}
tag to close the statement. The condition can be a simple boolean value, a comparison, or even a more complex expression involving variables and operators.
For example:
{% if user.is_authenticated %}
Welcome, {{ user.username }}!
{% else %}
Please log in to access this page.
{% endif %}
In this example, the if statement checks if the user is authenticated. If they are, it displays a welcome message with their username. If not, it displays a message asking them to log in.
Using If Statements with Variables and Operators
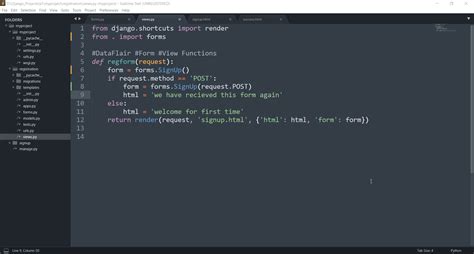
If statements in Django templates can also be used with variables and operators. You can compare values, check for equality, and even use logical operators like and
and or
.
For example:
{% if user.age > 18 and user.country == 'USA' %}
You are eligible to vote.
{% elif user.age > 18 and user.country != 'USA' %}
You are not eligible to vote in the USA.
{% else %}
You are not eligible to vote.
{% endif %}
In this example, the if statement checks two conditions: if the user's age is greater than 18 and if their country is the USA. If both conditions are true, it displays a message saying they are eligible to vote. If the first condition is true but the second is false, it displays a different message. If neither condition is true, it displays a third message.
Using If Statements with Lists and Tuples

If statements in Django templates can also be used with lists and tuples. You can check if a value is in a list or tuple, or if a list or tuple is empty.
For example:
{% if 'admin' in user.groups %}
You are an admin.
{% elif 'moderator' in user.groups %}
You are a moderator.
{% else %}
You are a regular user.
{% endif %}
In this example, the if statement checks if the string 'admin'
is in the user.groups
list. If it is, it displays a message saying the user is an admin. If the string 'moderator'
is in the list instead, it displays a different message. If neither string is in the list, it displays a third message.
Best Practices for Using If Statements in Django Templates
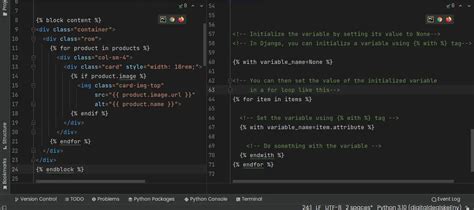
When using if statements in Django templates, there are several best practices to keep in mind. First, keep your if statements simple and easy to read. Avoid complex conditions and nested if statements, as they can make your template harder to understand and maintain.
Second, use meaningful variable names and comments to explain what your if statements are doing. This will make it easier for other developers to understand your code and for you to debug any issues that arise.
Finally, consider using template tags and filters to simplify your if statements and make your template more efficient. Django provides a range of built-in template tags and filters that can help you perform common tasks, such as checking if a value is empty or if a list contains a certain element.
Gallery of Django Template If Statement Examples
Django Template If Statement Examples


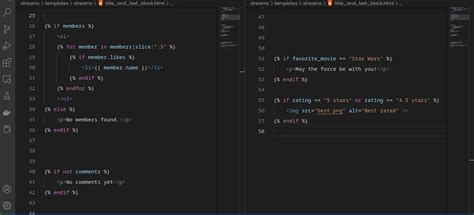




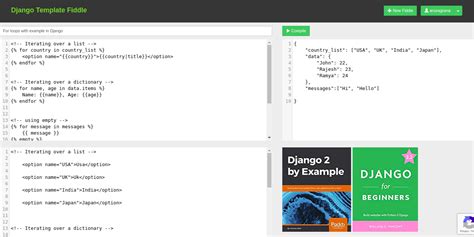
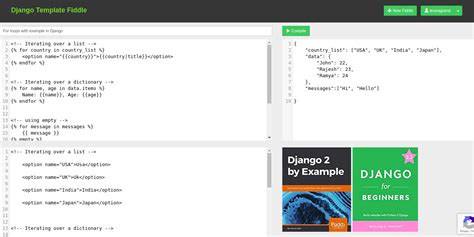

Frequently Asked Questions
What is the purpose of if statements in Django templates?
+If statements in Django templates are used to control the flow of the template based on conditions. They allow you to display or hide content, perform actions, and even loop through data.
How do I use if statements with variables and operators in Django templates?
+You can use if statements with variables and operators in Django templates by using the `{% if %}` tag and specifying the condition you want to evaluate. For example: `{% if user.age > 18 %}`.
What are some best practices for using if statements in Django templates?
+Some best practices for using if statements in Django templates include keeping your if statements simple and easy to read, using meaningful variable names and comments, and considering the use of template tags and filters to simplify your if statements.
In conclusion, if statements are a powerful tool in Django templates that allow you to create dynamic and interactive web pages. By understanding how to use if statements with variables, operators, and lists, you can build complex and efficient templates that adapt to different scenarios. Remember to keep your if statements simple, use meaningful variable names and comments, and consider using template tags and filters to simplify your code. With practice and experience, you'll become proficient in using if statements in Django templates and be able to build robust and scalable web applications. We hope this guide has been helpful in your journey to mastering Django template if statements. If you have any further questions or need more guidance, don't hesitate to reach out. Share your thoughts and experiences with if statements in Django templates in the comments below.