Intro
Learn Django template filter with multiple args, using filters like default, length, and truncate to customize template rendering with multiple arguments, enhancing template functionality.
Django template filters are a powerful tool for modifying and formatting data within templates. While many built-in filters accept only one argument, Django also provides a way to create custom filters that can handle multiple arguments, enhancing the flexibility and utility of your templates. In this article, we'll delve into the world of Django template filters, exploring how to use them, how they work, and most importantly, how to create custom filters that accept multiple arguments.
Template filters in Django are essentially functions that take one or more arguments and return a value that can be used directly within a template. They are applied using the pipe (|
) character after the variable or value you wish to modify. For example, the lower
filter converts a string to lowercase: {{ my_string|lower }}
. While this example uses a built-in filter with no arguments (beyond the variable being filtered), custom filters can be designed to accept additional arguments, allowing for more complex and dynamic data manipulation.
Why Use Custom Template Filters?
Before diving into the creation of custom filters, it's worth considering why they are useful. Built-in Django filters cover a wide range of common use cases, from formatting dates and numbers to truncating text and converting case. However, every project has unique requirements, and that's where custom filters come in. They allow developers to encapsulate specific logic or transformations that are repeatedly needed across the application, keeping templates clean and focused on presentation rather than complex data processing.
Creating a Custom Template Filter
Creating a custom template filter involves defining a Python function and then registering it with Django's template engine. Here's a basic example of a custom filter that accepts one argument:
# myapp/templatetags/my_filters.py
from django import template
register = template.Library()
@register.filter
def multiply(value, arg):
"""Multiply the value by the argument."""
return value * arg
To use this filter in a template, you would first load the custom filter library ({% load my_filters %}
), and then apply it to a value like so: {{ my_value|multiply:5 }}
.
Custom Filter with Multiple Arguments
While the previous example shows a filter with one additional argument, Django's filter system inherently supports passing multiple arguments by separating them with commas. For instance, if you wanted to create a filter that formats a string based on multiple parameters, you might do something like this:
# myapp/templatetags/my_filters.py
from django import template
register = template.Library()
@register.filter
def custom_format(value, arg1, arg2):
"""Format the value based on arg1 and arg2."""
# Example logic: Append arg1 and arg2 to value
return f"{value} - {arg1} - {arg2}"
You would use this filter in a template by passing the arguments separated by commas: {{ my_string|custom_format:"prefix","suffix" }}
.
Practical Example: Creating a Filter for Date Formatting
A common requirement in web development is formatting dates in a user-friendly way. While Django provides a date
filter for basic formatting, you might want a custom filter to handle more specific or complex date formatting based on multiple arguments (like the format string and a timezone).
# myapp/templatetags/date_filters.py
from django import template
from datetime import datetime
import pytz
register = template.Library()
@register.filter
def format_date(value, format_string, timezone_string):
"""Format a date based on the given format string and timezone."""
timezone = pytz.timezone(timezone_string)
localized_date = value.astimezone(timezone)
return localized_date.strftime(format_string)
To use this filter, you would load the date_filters
library and apply it to a date object, specifying the format and timezone: {{ my_date|format_date:"%d %B %Y","Europe/London" }}
.
Embedding Images
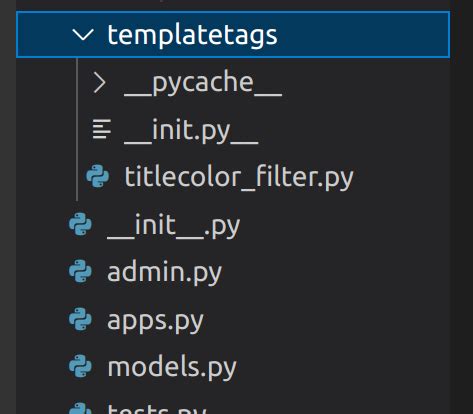
Gallery of Django Template Filters
Django Template Filter Gallery
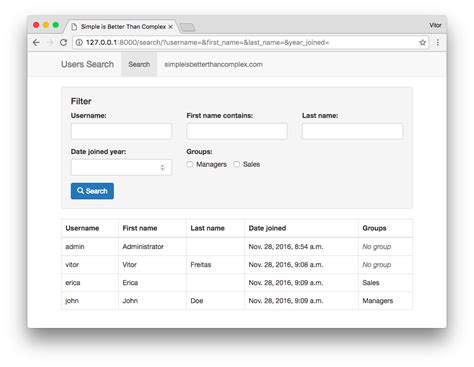
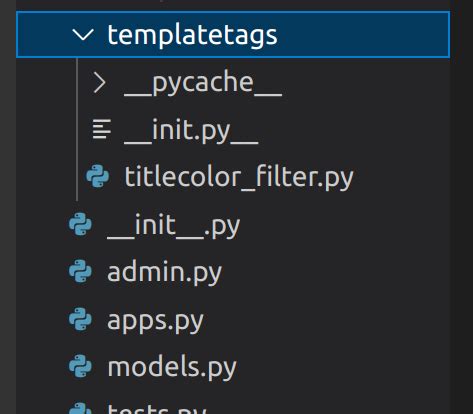
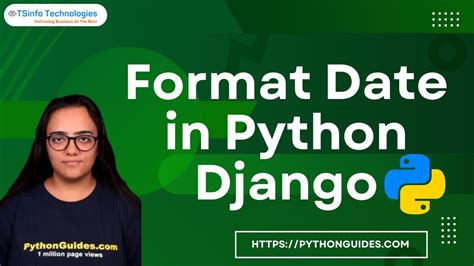
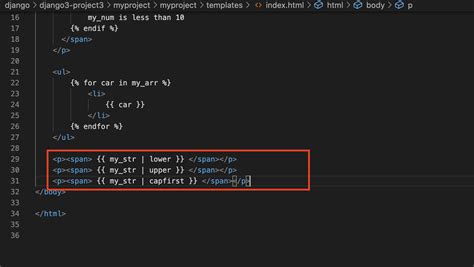
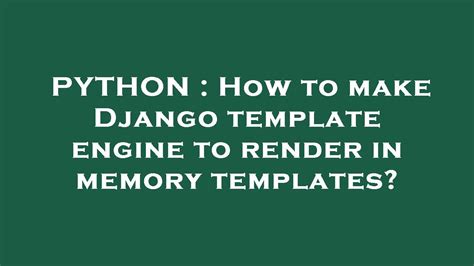
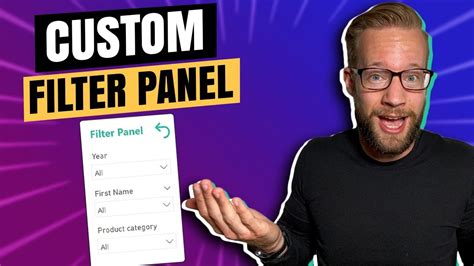
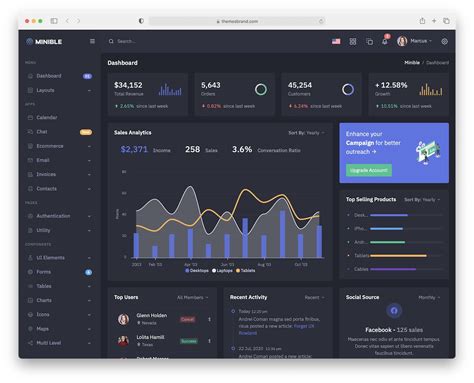
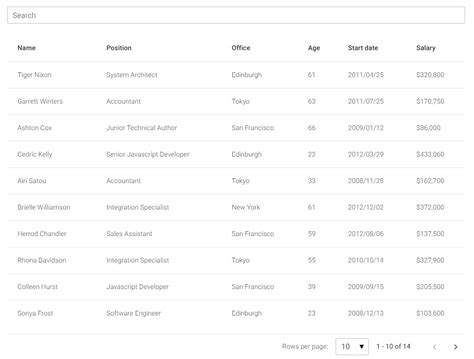
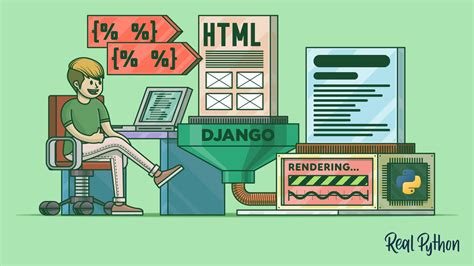
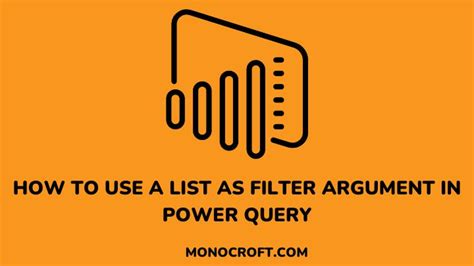
FAQs
How do I create a custom template filter in Django?
+To create a custom template filter, define a Python function in a file within a `templatetags` directory of your app, and register it with Django's template engine using `@register.filter`.
Can Django template filters accept multiple arguments?
+Yes, Django template filters can accept multiple arguments. These arguments are passed to the filter function separated by commas.
How do I use a custom template filter in my Django template?
+First, load the custom filter library at the top of your template with `{% load my_filters %}`, then apply the filter to a value using the pipe (`|`) character followed by the filter name and any arguments.
In conclusion, Django's template filter system is a versatile tool that can significantly enhance the functionality and readability of your templates. By understanding how to create and use custom filters, especially those that accept multiple arguments, you can tailor your templates to meet the unique needs of your application, making your development process more efficient and your code more maintainable. Whether you're working on a small project or a large-scale application, mastering custom template filters can take your Django development skills to the next level.